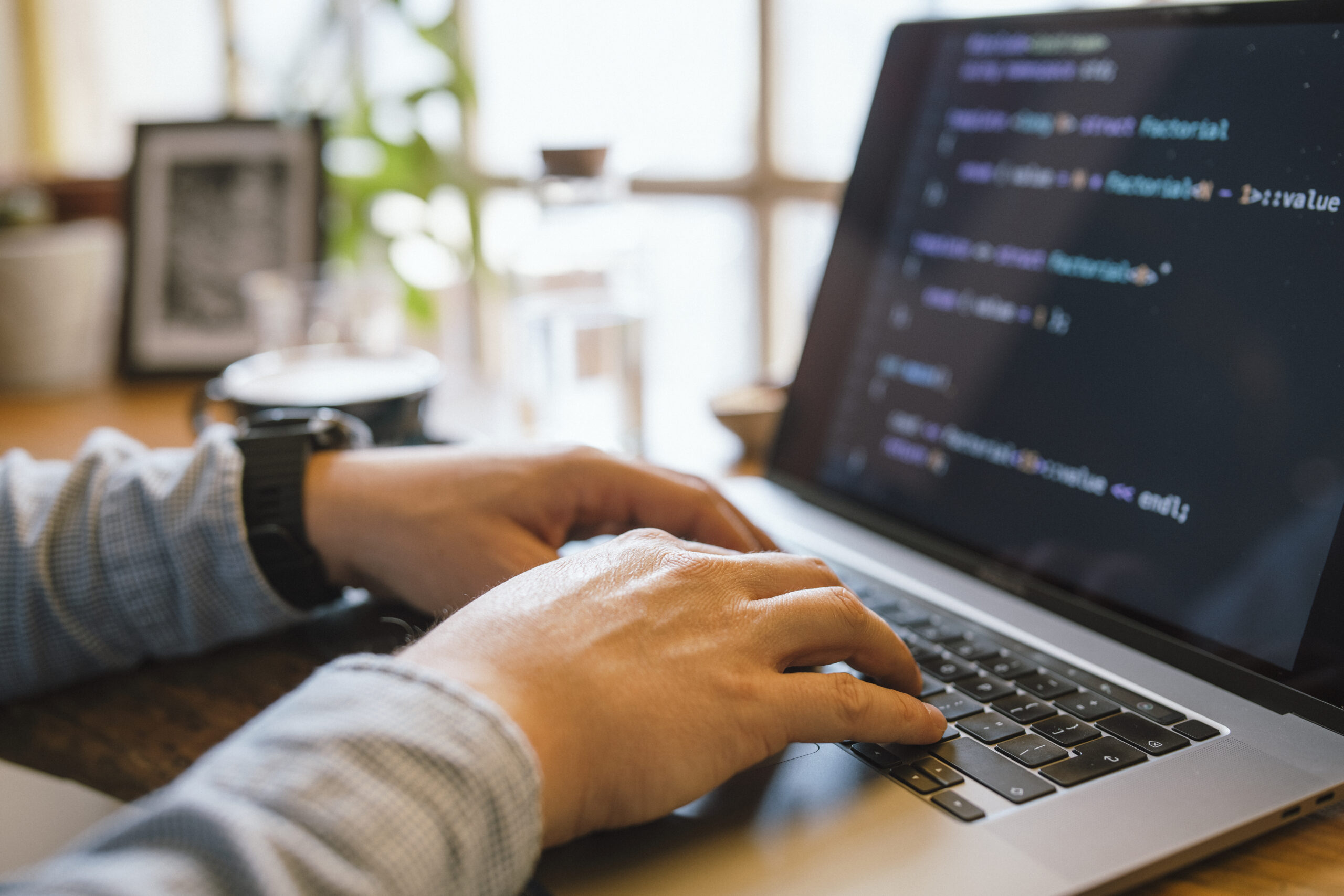
Debugging is Probably the most crucial — still normally overlooked — expertise in the developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about understanding how and why issues go Incorrect, and Understanding to Consider methodically to resolve troubles successfully. No matter if you are a rookie or maybe a seasoned developer, sharpening your debugging techniques can help save hrs of stress and substantially transform your productiveness. Allow me to share many techniques to aid developers amount up their debugging activity by me, Gustavo Woltmann.
Learn Your Equipment
One of many quickest ways builders can elevate their debugging capabilities is by mastering the equipment they use daily. Whilst crafting code is just one Section of advancement, understanding how to connect with it properly in the course of execution is Similarly significant. Modern day improvement environments occur Outfitted with powerful debugging abilities — but several builders only scratch the floor of what these tools can perform.
Consider, for example, an Built-in Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications allow you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code within the fly. When used correctly, they Enable you to notice specifically how your code behaves during execution, that's a must have for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-close developers. They help you inspect the DOM, keep track of network requests, watch genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can convert irritating UI difficulties into manageable jobs.
For backend or procedure-level builders, equipment like GDB (GNU Debugger), Valgrind, or LLDB present deep control above jogging procedures and memory management. Learning these equipment could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be snug with version Manage programs like Git to be aware of code history, discover the exact minute bugs were being released, and isolate problematic modifications.
In the end, mastering your equipment signifies likely beyond default settings and shortcuts — it’s about creating an intimate knowledge of your advancement surroundings to make sure that when issues arise, you’re not lost in the dark. The better you realize your resources, the more time it is possible to commit fixing the actual issue instead of fumbling via the method.
Reproduce the challenge
The most vital — and often ignored — steps in effective debugging is reproducing the issue. Prior to jumping into your code or building guesses, developers require to create a steady atmosphere or scenario wherever the bug reliably appears. With out reproducibility, correcting a bug will become a activity of probability, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a dilemma is accumulating as much context as possible. Talk to inquiries like: What actions brought about the issue? Which surroundings was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The greater detail you may have, the less difficult it turns into to isolate the precise problems under which the bug happens.
Once you’ve gathered sufficient information, endeavor to recreate the issue in your neighborhood environment. This might mean inputting precisely the same data, simulating identical consumer interactions, or mimicking process states. If the issue appears intermittently, take into consideration creating automatic exams that replicate the sting cases or condition transitions associated. These tests not merely assistance expose the situation but additionally protect against regressions Sooner or later.
In some cases, the issue could possibly be environment-particular — it might take place only on specified functioning systems, browsers, or beneath distinct configurations. Applying instruments like Digital equipment, containerization (e.g., Docker), or cross-browser testing platforms may be instrumental in replicating these kinds of bugs.
Reproducing the condition isn’t simply a step — it’s a way of thinking. It necessitates patience, observation, along with a methodical strategy. But as soon as you can continually recreate the bug, you might be currently halfway to fixing it. Having a reproducible situation, You can utilize your debugging equipment far more proficiently, test possible fixes safely, and communicate more clearly with your team or consumers. It turns an abstract complaint into a concrete obstacle — Which’s wherever builders thrive.
Examine and Fully grasp the Mistake Messages
Error messages tend to be the most respected clues a developer has when some thing goes Incorrect. Rather than viewing them as irritating interruptions, developers should really study to deal with error messages as immediate communications with the system. They normally inform you what exactly occurred, where it transpired, and often even why it occurred — if you know how to interpret them.
Start by examining the concept cautiously As well as in entire. Numerous builders, specially when beneath time pressure, look at the initial line and instantly get started generating assumptions. But deeper in the mistake stack or logs might lie the legitimate root result in. Don’t just duplicate and paste error messages into search engines like google — browse and have an understanding of them very first.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or perhaps a logic mistake? Does it issue to a particular file and line selection? What module or operate brought on it? These queries can guideline your investigation and level you towards the accountable code.
It’s also practical to comprehend the terminology of your programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Discovering to recognize these can substantially increase your debugging method.
Some glitches are imprecise or generic, and in People instances, it’s important to look at the context by which the error transpired. Check associated log entries, input values, and up to date adjustments while in the codebase.
Don’t ignore compiler or linter warnings either. These frequently precede more substantial challenges and supply hints about possible bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Studying to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a additional economical and self-assured developer.
Use Logging Wisely
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When utilised proficiently, it offers real-time insights into how an software behaves, helping you understand what’s happening underneath the hood without having to pause execution or move with the code line by line.
A great logging technique starts with knowing what to log and at what level. Common logging concentrations involve DEBUG, Facts, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic details in the course of improvement, INFO for typical situations (like effective start-ups), Alert for likely concerns that don’t break the applying, Mistake for real problems, and FATAL in the event the process can’t keep on.
Stay away from flooding your logs with excessive or irrelevant facts. Excessive logging can obscure crucial messages and decelerate your procedure. Center on crucial events, point out adjustments, enter/output values, and significant choice details within your code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and performance names, so it’s simpler to trace challenges in distributed methods or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all with no halting This system. They’re Specifically useful in output environments exactly where stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, wise logging is about stability and clarity. Which has a nicely-considered-out logging approach, more info you'll be able to lessen the time it takes to spot troubles, acquire deeper visibility into your purposes, and Increase the General maintainability and dependability within your code.
Think Just like a Detective
Debugging is not simply a technological activity—it is a method of investigation. To effectively recognize and correct bugs, builders will have to method the method similar to a detective resolving a secret. This state of mind aids break down advanced difficulties into workable parts and follow clues logically to uncover the basis bring about.
Get started by accumulating proof. Think about the indications of the problem: error messages, incorrect output, or overall performance concerns. The same as a detective surveys against the law scene, accumulate just as much suitable facts as you may without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer experiences to piece alongside one another a transparent photo of what’s occurring.
Up coming, type hypotheses. Request your self: What might be creating this conduct? Have any modifications lately been made to the codebase? Has this difficulty transpired ahead of below comparable instances? The goal is to slender down opportunities and recognize possible culprits.
Then, exam your theories systematically. Endeavor to recreate the challenge inside a managed setting. When you suspect a particular function or part, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the outcomes guide you nearer to the truth.
Pay back near attention to smaller particulars. Bugs normally conceal in the minimum expected places—just like a lacking semicolon, an off-by-one particular mistake, or possibly a race condition. Be extensive and patient, resisting the urge to patch The problem without entirely comprehension it. Temporary fixes may possibly disguise the real problem, just for it to resurface afterwards.
Finally, continue to keep notes on Everything you tried using and figured out. Just as detectives log their investigations, documenting your debugging approach can help you save time for potential difficulties and assist Some others comprehend your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical competencies, strategy complications methodically, and turn out to be simpler at uncovering concealed issues in sophisticated devices.
Write Assessments
Producing checks is one of the best tips on how to enhance your debugging expertise and Over-all enhancement performance. Tests not just aid capture bugs early but also serve as a safety net that gives you self confidence when building alterations on your codebase. A perfectly-analyzed software is much easier to debug as it means that you can pinpoint accurately where by and when a problem takes place.
Get started with device checks, which deal with unique capabilities or modules. These compact, isolated tests can rapidly reveal whether a specific bit of logic is Doing the job as predicted. Every time a examination fails, you quickly know in which to search, appreciably minimizing time invested debugging. Device checks are Specially valuable for catching regression bugs—concerns that reappear following previously staying fastened.
Up coming, integrate integration tests and close-to-conclusion assessments into your workflow. These assist ensure that many portions of your application work jointly easily. They’re notably helpful for catching bugs that manifest in intricate methods with multiple parts or solutions interacting. If a little something breaks, your assessments can tell you which Component of the pipeline failed and underneath what ailments.
Creating checks also forces you to Imagine critically about your code. To check a function appropriately, you'll need to be aware of its inputs, anticipated outputs, and edge scenarios. This degree of comprehension naturally qualified prospects to raised code construction and much less bugs.
When debugging an issue, composing a failing exam that reproduces the bug could be a robust first step. After the take a look at fails regularly, you can give attention to correcting the bug and watch your examination go when The difficulty is resolved. This technique makes certain that exactly the same bug doesn’t return Sooner or later.
To put it briefly, writing exams turns debugging from a aggravating guessing match right into a structured and predictable process—aiding you catch additional bugs, faster and much more reliably.
Just take Breaks
When debugging a difficult difficulty, it’s easy to become immersed in the situation—gazing your screen for hours, attempting Remedy soon after Option. But One of the more underrated debugging tools is simply stepping away. Taking breaks assists you reset your thoughts, lower annoyance, and infrequently see The difficulty from the new point of view.
When you are far too near to the code for way too very long, cognitive tiredness sets in. You would possibly start out overlooking evident glitches or misreading code that you choose to wrote just several hours before. With this condition, your brain turns into significantly less effective at issue-solving. A brief stroll, a coffee break, or even switching to a special job for 10–quarter-hour can refresh your emphasis. A lot of developers report finding the foundation of a challenge once they've taken time for you to disconnect, permitting their subconscious get the job done while in the track record.
Breaks also support avoid burnout, Particularly all through extended debugging periods. Sitting before a display, mentally trapped, is not simply unproductive but in addition draining. Stepping absent means that you can return with renewed Vitality along with a clearer mentality. You might quickly recognize a lacking semicolon, a logic flaw, or possibly a misplaced variable that eluded you prior to.
For those who’re caught, a very good guideline is to established a timer—debug actively for 45–60 minutes, then have a 5–ten moment crack. Use that time to maneuver close to, extend, or do one thing unrelated to code. It may well truly feel counterintuitive, Primarily below limited deadlines, nevertheless it basically results in a lot quicker and more effective debugging in the long run.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart tactic. It gives your brain Place to breathe, increases your viewpoint, and can help you steer clear of the tunnel vision That usually blocks your development. Debugging is actually a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Each and every bug you face is more than just A brief setback—It can be a possibility to develop like a developer. No matter if it’s a syntax mistake, a logic flaw, or a deep architectural challenge, every one can teach you some thing worthwhile when you take the time to reflect and evaluate what went Improper.
Start off by inquiring you a few important concerns after the bug is settled: What induced it? Why did it go unnoticed? Could it have been caught before with better practices like unit testing, code critiques, or logging? The answers frequently reveal blind places in your workflow or understanding and assist you to Develop stronger coding routines moving ahead.
Documenting bugs may also be a great behavior. Maintain a developer journal or maintain a log in which you Take note down bugs you’ve encountered, the way you solved them, and Whatever you realized. With time, you’ll start to see styles—recurring challenges or prevalent problems—which you could proactively stay away from.
In group environments, sharing what you've discovered from the bug with the peers may be especially impressive. No matter if it’s by way of a Slack message, a brief compose-up, or a quick know-how-sharing session, supporting Other people steer clear of the similar concern boosts team effectiveness and cultivates a more robust Understanding society.
Far more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial aspects of your growth journey. In the end, many of the greatest builders usually are not those who create great code, but people who consistently study from their errors.
Eventually, Every bug you deal with adds a whole new layer towards your skill established. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities takes time, apply, and tolerance — nevertheless the payoff is big. It will make you a more productive, self-confident, and able developer. The next time you are knee-deep in the mysterious bug, try to remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.